What are Functions and why do we need them?
Theoretically we do not really need functions … however, without functions our code would become super long and completely unreadable. Not to mention; we’d have to write a lot more code.
We have already worked with 2 functions that are required for your Arduino: “setup” and “loop”.
But what are functions and why do we need them? Or better said: why do we like them?
A function, is a group of instructions for a specific task.
When do we define our function?
- When code is repeated more than once in my program
- If my code becomes more readable with functions
- If my code becomes more manageable with functions
- If we’d like to re-use a piece of code for example in other programs
Let’s look at an example of what a function could be – just to grasp the concept.
Say we have a dog, which needs walking 4 times a day: At 8 AM, 12 PM, 5 PM, and at 9 PM.
The task of walking the dog involves:
– Put on a coat
– Put on the leash of the dog
– Go outside
– Walk through the park for 15 minutes
– Go back inside
– Take off the leash of the dog
– Take off your coat.
Now let’s assume our program handles our day when it comes to walking the dog:
if 8 AM then
– Put on a coat
– Put on the leash of the dog
– Go outside
– Walk through the park for 15 minutes
– Go back inside
– Take off the leash of the dog
– Take off your coat.
if 12 PM then
– Put on a coat
– Put on the leash of the dog
– Go outside
– Walk through the park for 15 minutes
– Go back inside
– Take off the leash of the dog
– Take off your coat.
if 5 PM then
– Put on a coat
– Put on the leash of the dog
– Go outside
– Walk through the park for 15 minutes
– Go back inside
– Take off the leash of the dog
– Take off your coat.
if 9 PM then
– Put on a coat
– Put on the leash of the dog
– Go outside
– Walk through the park for 15 minutes
– Go back inside
– Take off the leash of the dog
– Take off your coat.
Our program is pretty long, right? And a lot of repeating code as well …
We could create a function, let’s call it “WalkTheDog()”, and define it as follows.
WalkTheDog():
– Put on a coat
– Put on the leash of the dog
– Go outside
– Walk through the park for 15 minutes
– Go back inside
– Take off the leash of the dog
– Take off your coat.
Now our program would look much simpler:
if 8 AM then
WalkTheDog()
if 12 PM then
WalkTheDog()
if 5 PM then
WalkTheDog()
if 9 PM then
WalkTheDog()
You see, that not only our code has gotten much shorter, but it also has become much more readable – the “function” is called as if it was a regular statement. We in fact expanded our “language”.
In most scenario’s, using your own functions will also result in a smaller compiled program for your Arduino or computer, therefor being more efficient with memory needed on your Arduino (or computer).
But functions have also the advantage that if you made a mistake in your steps of walking the dog, that you only have to modify your code in one spot: the function – which makes your code more manageable. For example, if we forgot to add “Unlock the door” as a step. We just edit the function WalkTheDog() instead of having to edit the code in 4 different spots in the previous code.
Now if we have a function that is more of a generic use, we can even put them in what is called a library and re-use the function in our other programs.
Please keep in mind that:
- A function is like a little program on it’s own … inside a program
- A function can have functions inside it …
- A function has a “scope” – like we have seen with variables.
Creating our own Function(s)
It’s pretty easy to define our own functions in the language C which we use for Arduino programming. The basic structure looks like this:
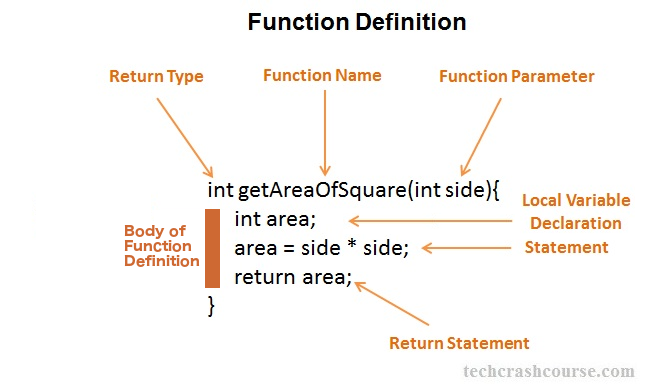
Obviously, our function needs a Function Name, and we need to follow the same naming rules as with variables and constants:
A function name:
- Should start with a letter (a, b, …, z, A, B, … , Z) or underscore ( _ )
- Should be descriptive of what the function
- Can contain letters, numbers, or underscore(s)
- CAN NOT contain special characters, symbols or spaces
- is case sensitive!
The Function Parameters are zero or more values that we want to pass to the function. The number of values and the datatype of these values however is fixed and defined in our function definition!
We have used function parameters before, even though you might not have been fully aware. It’s those values we pass between brackets, for example in this line: Serial.print("Hello");
Here we call the function “print()” from the object “Serial” and we pass the parameter “Hello” (a string). More about objects later.
Let’s start with a simple function:
1
2 3 |
void SayHello() {
Serial.println("Hello"); } |
This function, called “SayHello()”, takes no parameters since there is nothing between the () parentheses.
It also does not return a value, since we used the special datatype “void” – which means “nothing, thin air, nada, zilcho, niet, bupkis”.
The “body” of the function (the code block), holds the instructions for this function, and it just outputs “Hello”.
An example of how we’d use this:
1
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
void setup() {
// set the speed for the serial monitor: Serial.begin(9600); for(int count = 1; count <= 5; count++) { SayHello(); } } void loop() { // leave empty for now } void SayHello() { Serial.println("Hello"); } |
Most of this should look familiar, I’d hope anyway …
At the end of the code you see how we defined our “SayHello()” function.
In the “for”-loop you see how we call our function 5 times, which results in an output like this:
Hello
Hello
Hello
Hello
Hello
Feel free to insert Adele reference here (or Lionel Ritchie for the retro types).
The above guide was adapted by Mr. Stewart from this website.
Check Your Understanding
1. To create a function named blinkAllLeds with no parameters or return values, open the function (think of the dictionary) with the statement (line of code) (don't forgot the symbols, use spacing as above examples).
2. To use this new function blinkAllLeds, make a to it using the statement (line of code) (no spaces).
Next Page